[discord.py] グローバルチャットをつくる (Webhook版)
この記事について
この記事ではdiscord.pyを使って、Webhook版のグローバルチャットを作成します。
PythonやBotの稼働環境などは既に構築済みのものとして話を進めます。
また、前の記事で説明していたイベント云々の機能・仕組みを理解している前提です。
Webhookをつくろう
Webhookを作成しないことにはまず始まりません。
チャンネルの設定からも作成できますが、どうせならBotから作成しちゃいましょう
今回からはコマンドフレームワークを使用します。
1
2
3
4
5
6
7
8
9
10
11
12
13
|
import discord
from discord.ext import commands
# Botオブジェクト作成
bot = commands.Bot(command_prefix="!", intents=discord.Intents.all())
bot.GLOBALCHAT_NAME = "globalchat" # グローバルチャットの名前
bot.webhooks = {} # !create で作成したWebhookをおいておく場所
@bot.command()
async def create(ctx: commands.Context):
webhook = await ctx.channel.create_webhook(name="globalchat")
bot.webhooks[ctx.guild.id] = webhook.url
return await ctx.reply("> 作成しました! {0.url}".format(webhook))
|
これで !create
をチャンネルで入力すると、権限不足でなければWebhookが作成されます
が、Webhookを保存しておかなければ後々使えませんので、どこかに置いておきましょう
Ex: bot.webhooks = {}
にしておいてその中に足していく等
メッセージ送受信
さっき作ったWebhookを使います
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
|
@bot.event
async def on_message(msg: discord.Message):
# メッセージを送信したユーザーがBotならreturn
if msg.author.bot:
return
if msg.author.id == bot.user.id:
return
# チャンネルがグローバルチャットでなければreturn
if not msg.channel.name == bot.GLOBALCHAT_NAME:
return
# このコードではメッセージを送信したチャンネルにもwebhookが送信されるので、
# 邪魔な元のメッセージは消しておく
await msg.delete()
for i in bot.webhooks.values():
# Webhookオブジェ作成
wh = discord.Webhook.from_url(i)
# メッセージに画像が添付されているか
if msg.attachments:
await wh.send(
content=msg.content, # メッセージの内容
username=msg.author, # ユーザー名設定
avatar_url=msg.author.avatar, # アイコン設定
files=[await i.to_file() for i in msg.attachments], # 画像とか
allowed_mentions=discord.AllowedMentions.none() # メンション無効化
)
else:
await wh.send(
content=msg.content, # メッセージの内容
username=msg.author, # ユーザー名設定
avatar_url=msg.author.avatar, # アイコン設定
allowed_mentions=discord.AllowedMentions.none() # メンション無効化
)
|
こんな感じで動くと思います ↓↓↓

これをいじって自分好みのものにしてみましょう!
※2025年4月時点で、動作するとは限りません
おわり
前回同様この記事内のコードでは動作しない場合や、思っているような動作にはならない場合があります。
宣伝
こういうBot作ってます↓↓↓
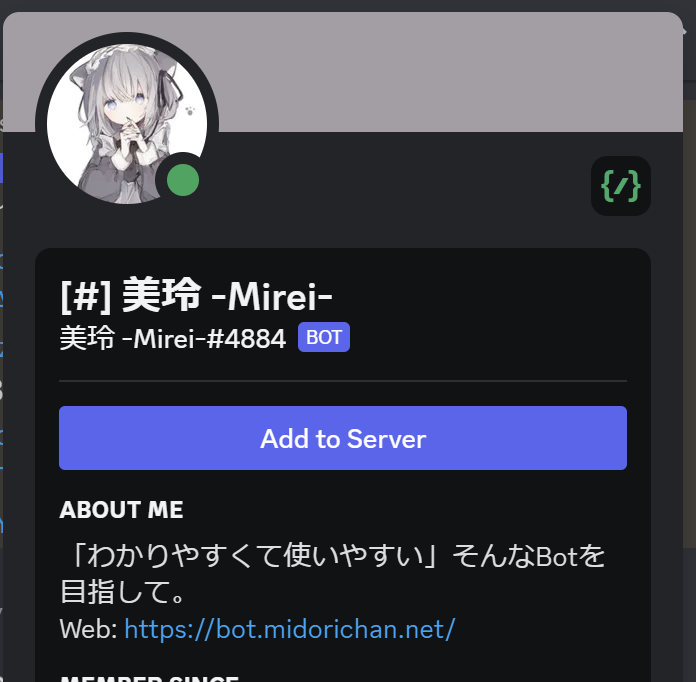
Bot Webサイト |
導入はこちら